New GD Functions
In PHP 7.2, four new functions were added to the gd
extension.
With imagesetclip()
, you can define a clipping area.
A clipping area limits where you can draw to the image, meaning that
you cannot draw outside the clipping area. The corresponding
function imagegetclip()
returns the upper left and
lower right corner of the currently defined clipping area. By
default, the clipping area is the whole image.
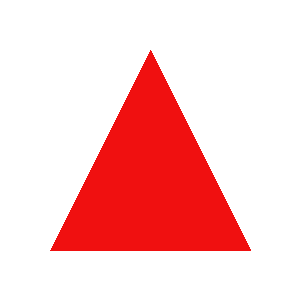
To play with clipping, let us first create an image with a red triangle on a white background:
$image = imagecreatetruecolor(300, 300);
$white = imagecolorallocate($image, 255, 255, 255);
imagefill ($image, 0, 0, $white);
$red = imagecolorallocate($image, 240, 16, 16);
imagefilledpolygon($image, [150, 50, 50, 250, 250, 250], 3, $red);
imagepng($image, 'triangle.png');
Now let us define a clipping area before we draw the triangle:
$image = imagecreatetruecolor(300, 300);
var_dump(imagegetclip($image));
$white = imagecolorallocate($image, 255, 255, 255);
imagefill ($image, 0, 0, $white);
imagesetclip($image, 100, 100, 200, 200);
var_dump(imagegetclip($image));
$red = imagecolorallocate($image, 240, 16, 16);
imagefilledpolygon($image, [150, 50, 50, 250, 250, 250], 3, $red);
imagepng($image, 'clipped-triangle.png');
imagedestroy($image);
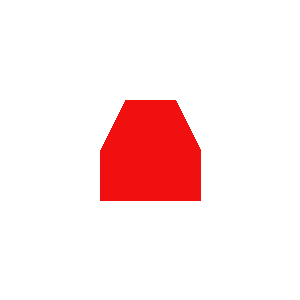
This script will also produce the following output:
array(4) {
[0] => int(0)
[1] => int(0)
[2] => int(299)
[3] => int(299)
}
array(4) {
[0] => int(100)
[1] => int(100)
[2] => int(200)
[3] => int(200)
}
As we can see, the clipping area initially is the whole image,
then it gets reduced in size as we set it using
imagesetclip()
.
The imageopenpolygon()
function draws an open
polygon, meaning that the first and last point will not be
connected. Let us modify the program creating the red triangle to
use imageopenpolygon()
:
$image = imagecreatetruecolor(300, 300);
$white = imagecolorallocate($image, 255, 255, 255);
imagefill ($image, 0, 0, $white);
$red = imagecolorallocate($image, 240, 16, 16);
imageopenpolygon($image, [150, 50, 50, 250, 250, 250], 3, $red);
imagepng($image, 'open-polygon.png');
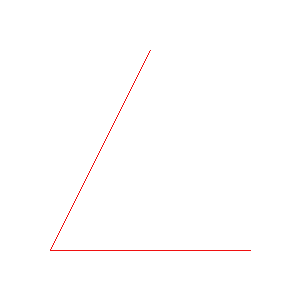
Using imageresolution()
, you can query and set the
image resolution, which is measured in dots per inch.
$image = imagecreatetruecolor(300, 300);
var_dump(imageresolution($image));
imageresolution($image, 150, 150);
var_dump(imageresolution($image));
This program will produce the following output:
array(2) {
[0] => int(96)
[1] => int(96)
}
array(2) {
[0] => int(150)
[1] => int(150)
}
As you can see, the default resolution is 96 dots per inch. Please note that the resolution is just metadata that will be written to the image file. It will not affect any of the drawing operations.